Python- Type Casting
Let’s dive deep into typecasting in Python, exploring its nuances, types, and practical applications. The article will cover the following sections:
- Introduction to Typecasting
- Why Typecasting is Important
- Types of Typecasting in Python
- Explicit Typecasting
- Implicit Typecasting
- Common Typecasting Functions
- Typecasting in Built-in Data Types
- Integers and Floats
- Strings
- Lists, Tuples, and Sets
- Dictionaries
- Practical Examples and Use Cases
- Common Errors and Pitfalls
- Best Practices for Typecasting
- Conclusion
1. Introduction to Typecasting
Typecasting, also known as type conversion, is the process of converting one data type into another. In Python, this can be done automatically (implicit typecasting) or manually (explicit typecasting). Understanding how and when to use typecasting is crucial for effective and efficient programming.
2. Why Typecasting is Important
Python is a dynamically typed language, meaning that variables do not need to declare their data type. However, different operations often require data to be in a specific type. Typecasting ensures that data types are appropriately converted, allowing for smooth execution of operations and functions.
For instance, consider a scenario where you want to concatenate a string with a number. Directly concatenating them will result in a TypeError, but typecasting can help resolve this issue.
3. Types of Typecasting in Python
Explicit Typecasting
Explicit typecasting is when a developer manually converts one data type into another. This is done using Python’s built-in functions, such as int()
, float()
, str()
, etc. Explicit typecasting requires careful attention to ensure data is converted correctly without loss or unintended consequences.
Implicit Typecasting
Implicit typecasting, on the other hand, is automatically performed by Python. The interpreter converts the data type without the programmer’s intervention, typically during arithmetic operations. This occurs when Python converts a smaller data type (like an integer) to a larger data type (like a float) to prevent data loss.
4. Common Typecasting Functions
Python provides several built-in functions for explicit typecasting:
int()
: Converts a value to an integer.float()
: Converts a value to a float.str()
: Converts a value to a string.list()
: Converts a value to a list.tuple()
: Converts a value to a tuple.set()
: Converts a value to a set.dict()
: Converts a value to a dictionary.
Each function has specific rules and behavior, which we will explore further.
5. Typecasting in Built-in Data Types
Integers and Floats
Integers and floats are fundamental numeric types in Python. Typecasting between these types is common in arithmetic operations. For example, dividing two integers may require one to be converted into a float to avoid integer division.
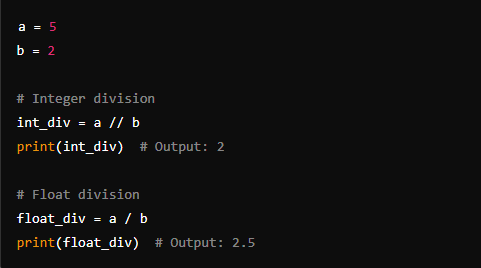
In the above example, dividing a
and b
as integers results in integer division, which discards the decimal part. By converting the result to a float, we preserve the decimal.
Strings
Typecasting to and from strings is often necessary when dealing with user input, file I/O, or data serialization. Converting numbers or other data types into strings allows them to be concatenated, displayed, or stored in a text format.

Here, the integer age
is converted into a string to concatenate it with another string.
Lists, Tuples, and Sets
Converting between lists, tuples, and sets can be useful for changing data mutability or removing duplicates.

Dictionaries
Typecasting into dictionaries is not as straightforward, as dictionaries require key-value pairs. However, it is possible to convert lists of tuples or other iterable objects into dictionaries.

6. Practical Examples and Use Cases
Example 1: User Input
User input is often taken as a string, but may need to be converted into integers or floats for calculations.

Here, the input string is converted into an integer for mathematical operations.
Example 2: Data Processing
In data processing, converting data types can help ensure data integrity and proper manipulation.

Using map()
, we convert a list of strings into a list of integers.
Example 3: Handling None Values
Typecasting can also help manage None
values, especially in functions that expect specific data types.

In this example, None
is handled by providing a default value.
7. Common Errors and Pitfalls
Typecasting can lead to errors if not done correctly. Common issues include:
- ValueError: Occurs when a value cannot be converted to the target type.
- TypeError: Happens when incompatible types are used in operations.
- Data Loss: Converting from float to int truncates the decimal part.
Example of ValueError:

8. Best Practices for Typecasting
- Always Validate Input: Before typecasting, ensure that the input can be safely converted.
- Handle Exceptions: Use try-except blocks to handle potential errors during typecasting.
- Be Mindful of Data Loss: Be aware of potential data loss, especially when converting between numeric types.
- Use Explicit Typecasting: Prefer explicit typecasting to avoid unexpected behavior from implicit conversions.
9. Conclusion
Typecasting is a fundamental aspect of Python programming, allowing for flexibility and robustness in handling different data types. Whether you’re dealing with user input, data processing, or mathematical computations, understanding and using typecasting correctly is essential. By following best practices and being aware of potential pitfalls, you can ensure your programs are reliable and error-free.