Python, a powerful and versatile programming language, is widely used in various domains such as web development, data analysis, artificial intelligence, and scientific computing. One of the fundamental aspects of Python is its handling of data through different data types. In this comprehensive guide, we’ll delve into Python’s data types, providing examples and explanations to help you understand their usage and significance.
1. Introduction to Data Types
Data types are essential in programming as they define the nature of the data that can be stored and manipulated within a program. In Python, data types are categorized into several main types:
- Numeric Types: int, float, complex
- Sequence Types: list, tuple, range
- Text Type: str
- Binary Types: bytes, bytearray, memoryview
- Mapping Type: dict
- Set Types: set, frozenset
- Boolean Type: bool
- None Type: NoneType
Let’s explore each of these data types in detail.
2. Numeric Types
a. Integer (int)
Integers are whole numbers, both positive and negative, without any decimal point. In Python, integers can be of arbitrary precision, meaning they can be as large as the memory allows.
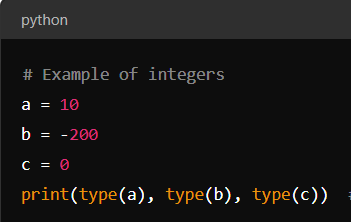
b. Float
Floats are numbers with a decimal point. They are used to represent real numbers.
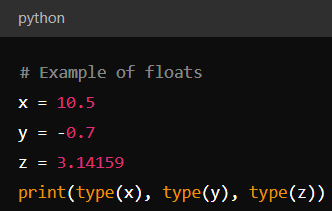
c. Complex
Complex numbers have a real and an imaginary part, which are represented as a + bj
where a
is the real part and b
is the imaginary part.
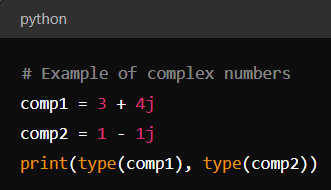
3. Sequence Types
a. List
Lists are ordered, mutable collections of items. They can store elements of different data types.

b. Tuple
Tuples are ordered, immutable collections of items. Like lists, they can store elements of different data types.

. Range
Ranges represent an immutable sequence of numbers, commonly used for looping a specific number of times in for-loops.
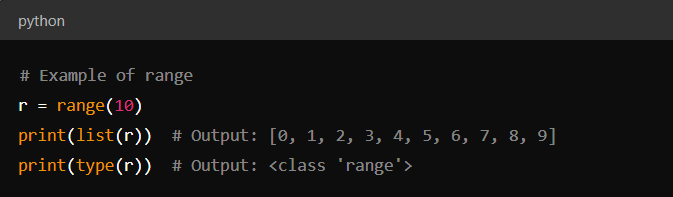
4. Text Type
a. String (str)
Strings are sequences of characters enclosed within single, double, or triple quotes.

5. Binary Types
a. Bytes
Bytes are immutable sequences of bytes, often used for binary data.

b. Bytearray
Bytearrays are mutable sequences of bytes.

c. Memoryview
Memoryview objects allow Python code to access the internal data of an object that supports the buffer protocol without copying.

6. Mapping Type
a. Dictionary (dict)
Dictionaries are unordered, mutable collections of key-value pairs. They are indexed by keys, which can be any immutable type.

7. Set Types
a. Set
Sets are unordered collections of unique items. They are mutable and do not allow duplicate elements.
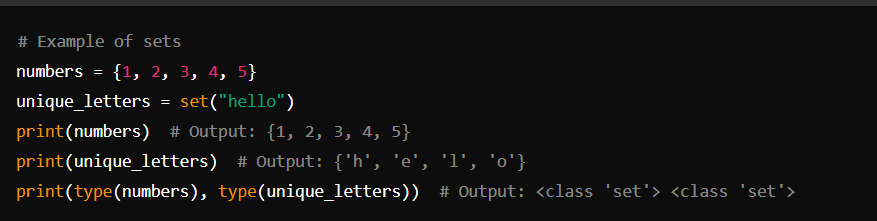
b. Frozenset
Frozensets are immutable versions of sets.

8. Boolean Type
a. Boolean (bool)
Booleans represent one of two values: True or False.

9. None Type
a. NoneType
NoneType has a single value, None, which is used to signify the absence of a value or a null value.

10. Conclusion
Understanding Python’s data types is crucial for effective programming. Each type serves a specific purpose, and knowing when and how to use them can greatly enhance your coding efficiency and clarity. From basic numeric and text types to more complex collections like lists, dictionaries, and sets, Python provides a rich set of tools for handling data. Whether you are a beginner or an experienced programmer, mastering these data types is a foundational step in your Python journey.
In this guide, we’ve covered the essential data types in Python with examples to illustrate their usage. As you continue to explore and practice Python, you’ll find these data types indispensable in writing clean, efficient, and powerful code.