Python Operators - Introduction
In Python, operators are special symbols or keywords that perform operations on values or variables. These operations range from arithmetic calculations to comparisons, logical operations, and more. Understanding how these operators work is fundamental to writing effective Python code.
1. Arithmetic Operators
Arithmetic operators are used to perform mathematical operations such as addition, subtraction, multiplication, and division.
+
: Addition- Adds two operands.
- Example:
3 + 2
results in5
.
-
: Subtraction- Subtracts the second operand from the first.
- Example:
5 - 2
results in3
.
*
: Multiplication- Multiplies two operands.
- Example:
3 * 2
results in6
.
/
: Division- Divides the first operand by the second. Returns a float.
- Example:
5 / 2
results in2.5
.
%
: Modulus- Returns the remainder of the division.
- Example:
5 % 2
results in1
.
**
: Exponentiation- Raises the first operand to the power of the second.
- Example:
2 ** 3
results in8
.
//
: Floor Division- Divides the first operand by the second and returns the largest integer less than or equal to the result.
- Example:
5 // 2
results in2
.
2. Comparison Operators
Comparison operators are used to compare two values. They return either True
or False
.
==
: Equal- Checks if two operands are equal.
- Example:
5 == 5
results inTrue
.
!=
: Not Equal- Checks if two operands are not equal.
- Example:
5 != 2
results inTrue
.
>
: Greater Than- Checks if the left operand is greater than the right.
- Example:
5 > 2
results inTrue
.
<
: Less Than- Checks if the left operand is less than the right.
- Example:
2 < 5
results inTrue
.
>=
: Greater Than or Equal To- Checks if the left operand is greater than or equal to the right.
- Example:
5 >= 5
results inTrue
.
<=
: Less Than or Equal To- Checks if the left operand is less than or equal to the right.
- Example:
2 <= 5
results inTrue
.
3. Logical Operators
Logical operators are used to combine conditional statements.
and
: Logical AND- Returns
True
if both statements are true. - Example:
(5 > 2) and (3 < 4)
results inTrue
.
- Returns
or
: Logical OR- Returns
True
if at least one statement is true. - Example:
(5 > 2) or (3 > 4)
results inTrue
.
- Returns
not
: Logical NOT- Reverses the result, returns
False
if the result is true. - Example:
not(5 > 2)
results inFalse
.
- Reverses the result, returns
4. Assignment Operators
Assignment operators are used to assign values to variables.
=
: Assign- Assigns a value to a variable.
- Example:
x = 5
assigns5
tox
.
+=
: Add and Assign- Adds the right operand to the left operand and assigns the result to the left operand.
- Example:
x += 3
is equivalent tox = x + 3
.
-=
: Subtract and Assign- Subtracts the right operand from the left operand and assigns the result to the left operand.
- Example:
x -= 3
is equivalent tox = x - 3
.
*=
: Multiply and Assign- Multiplies the left operand with the right operand and assigns the result to the left operand.
- Example:
x *= 3
is equivalent tox = x * 3
.
/=
: Divide and Assign- Divides the left operand by the right operand and assigns the result to the left operand.
- Example:
x /= 3
is equivalent tox = x / 3
.
%=
: Modulus and Assign- Takes modulus using two operands and assigns the result to the left operand.
- Example:
x %= 3
is equivalent tox = x % 3
.
**=
: Exponent and Assign- Raises the left operand to the power of the right operand and assigns the result to the left operand.
- Example:
x **= 3
is equivalent tox = x ** 3
.
//=
: Floor Divide and Assign- Performs floor division on two operands and assigns the result to the left operand.
- Example:
x //= 3
is equivalent tox = x // 3
.
5. Bitwise Operators
Bitwise operators work on bits and perform bit-by-bit operations.
&
: Bitwise AND- Sets each bit to 1 if both bits are 1.
- Example:
5 & 3
results in1
.
|
: Bitwise OR- Sets each bit to 1 if one of two bits is 1.
- Example:
5 | 3
results in7
.
^
: Bitwise XOR- Sets each bit to 1 if only one of two bits is 1.
- Example:
5 ^ 3
results in6
.
~
: Bitwise NOT- Inverts all the bits.
- Example:
~5
results in-6
.
<<
: Bitwise Left Shift- Shifts bits to the left by the number of positions specified.
- Example:
5 << 1
results in10
.
>>
: Bitwise Right Shift- Shifts bits to the right by the number of positions specified.
- Example:
5 >> 1
results in2
.
6. Membership Operators
Membership operators are used to test if a sequence contains an element.
in
: Checks if a value is in a sequence.- Example:
3 in [1, 2, 3]
results inTrue
.
- Example:
not in
: Checks if a value is not in a sequence.- Example:
4 not in [1, 2, 3]
results inTrue
.
- Example:
7. Identity Operators
Identity operators are used to compare the memory locations of two objects.
is
: Checks if two variables point to the same object.- Example:
x is y
results inTrue
ifx
andy
are the same object.
- Example:
is not
: Checks if two variables do not point to the same object.- Example:
x is not y
results inTrue
ifx
andy
are not the same object.
- Example:
8. Special Operators
:=
: Walrus Operator- Used for assignment expressions. It assigns values to variables as part of an expression.
- Example:
(n := len(x)) > 10
assignslen(x)
ton
and then compares it with10
.
Example
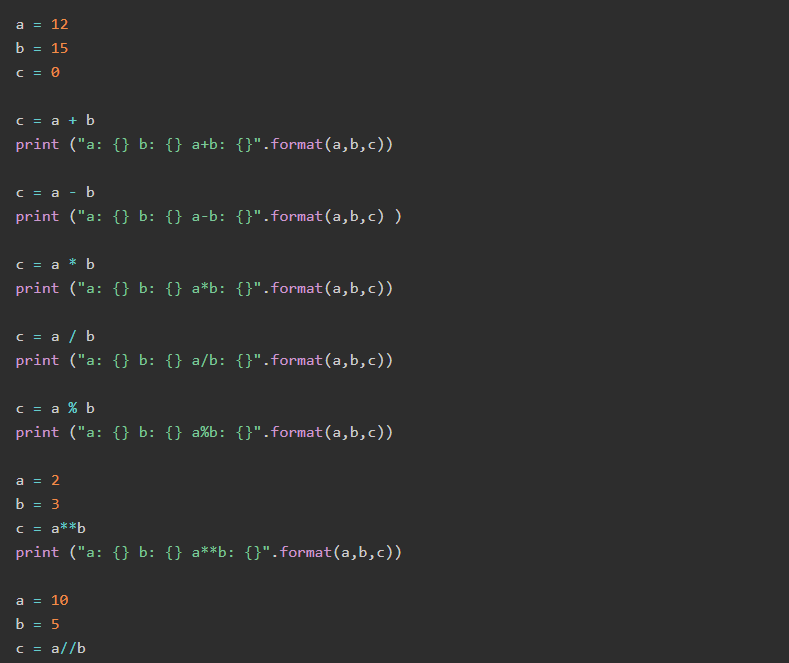
Output
